Make use of Google Earth's detailed globe by tilting the map to save a perfect 3D view or diving into Street View for a 360 experience. Share your story with the world. The long string is simply a binary sequence converted to ASCII. The first for statement makes b start out at 10, and the b21 after the string yields 31. Treating the string as an array, offset 31 is the start of the 'real' data in the string (the second line in the code sample you provided).
- C Program To Print World Map
- C Program To Print World Map Pdf
- C Program To Print World Map Worksheet
- C Program To Print World Map Online
This post will discuss how to print out all keys and values from a std::map
or std::unordered_map
in C++.
Maps are associative containers whose elements are key-value pairs. Using a map, we can associate a value with some key and later retrieve that value using the same key. The retrieval operation in a map is very fast. There are several ways in C++ to print out all pairs present on the map:
1. Using range-based for-loop
The recommended approach in C++11 is to use the new range-based for-loops for printing the map pairs, as shown below:
2 4 6 8 10 12 14 16 18 20 22 | #include <unordered_map> template<typenameK,typenameV> { std::cout<<'{'<<pair.first<<': '<<pair.second<<'}n'; } intmain() std::unordered_map<int,char>m={ {2,'B'}, }; } |
Output:
{3: C}
{1: A}
{2: B}
2. Using std::for_each
function
Another simple solution is to use std::for_each
. It takes an iterator to the beginning and end of the map, and applies a specified function on every pair within that range. The specified function may be a unary function, a lambda expression, or an object of a class overloading the ()
operator.
2 4 6 8 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40 42 44 46 | #include <unordered_map> { voidoperator()(conststd::pair<K,V>&p){ } voidprint(conststd::pair<K,V>&p){ } template<typenameK,typenameV> { std::for_each(m.begin(), [](conststd::pair<int,char>&p){ }); // or pass an object of a class overloading the ()operator // std::for_each(m.begin(), m.end(), print<K, V>); { {1,'A'}, {3,'C'} } |
Output:
{3: C}
{1: A}
{2: B}
3. Using Iterator
We can also use iterators to iterate a map and print its pairs. It is recommended to use the const_iterator
from C++11, as we’re not modifying the map pairs inside the for-loop.
2 4 6 8 10 12 14 16 18 20 22 | #include <unordered_map> template<typenameK,typenameV> { std::cout<<'{'<<(*it).first<<': '<<(*it).second<<'}n'; } intmain() std::unordered_map<int,char>m={ {2,'B'}, }; } |
Output:
{3: C}
{1: A}
{2: B}
4. Operator<< Overloading
To get std::cout
to accept the map object, we can overload the <<
operator to recognize an ostream object on the left and a map object on the right, as shown below:
2 4 6 8 10 12 14 16 18 20 22 24 | #include <unordered_map> template<typenameK,typenameV> conststd::unordered_map<K,V>&m){ os<<'{'<<p.first<<': '<<p.second<<'}n'; returnos; { {1,'A'}, {3,'C'} } |
Output:
{3: C}
{1: A}
{2: B}
That’s all about printing out all keys and values from a map in C++.
Objective

In this challenge, we will learn some basic concepts of C that will get you started with the language. You will need to use the same syntax to read input and write output in many C challenges.
Task
This challenge requires you to print Hello, World on a single line, and then print the already provided input string to stdout.
Note: You do not need to read any input in this challenge.
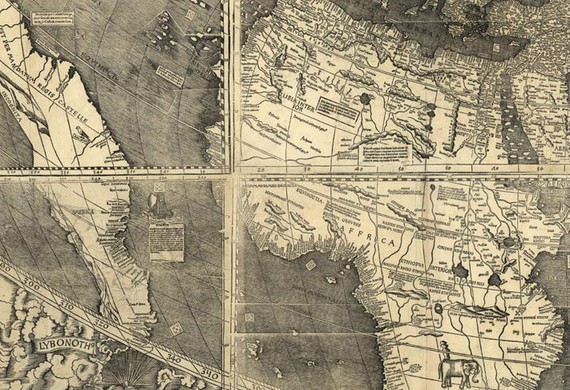
C Program To Print World Map
Input Format
You do not need to read any input in this challenge.
C Program To Print World Map Pdf
Output Format
C Program To Print World Map Worksheet
Print Hello, World on the first line, and the string from the given input on the second line.
Sample Input 0
C Program To Print World Map Online
Sample Output 0
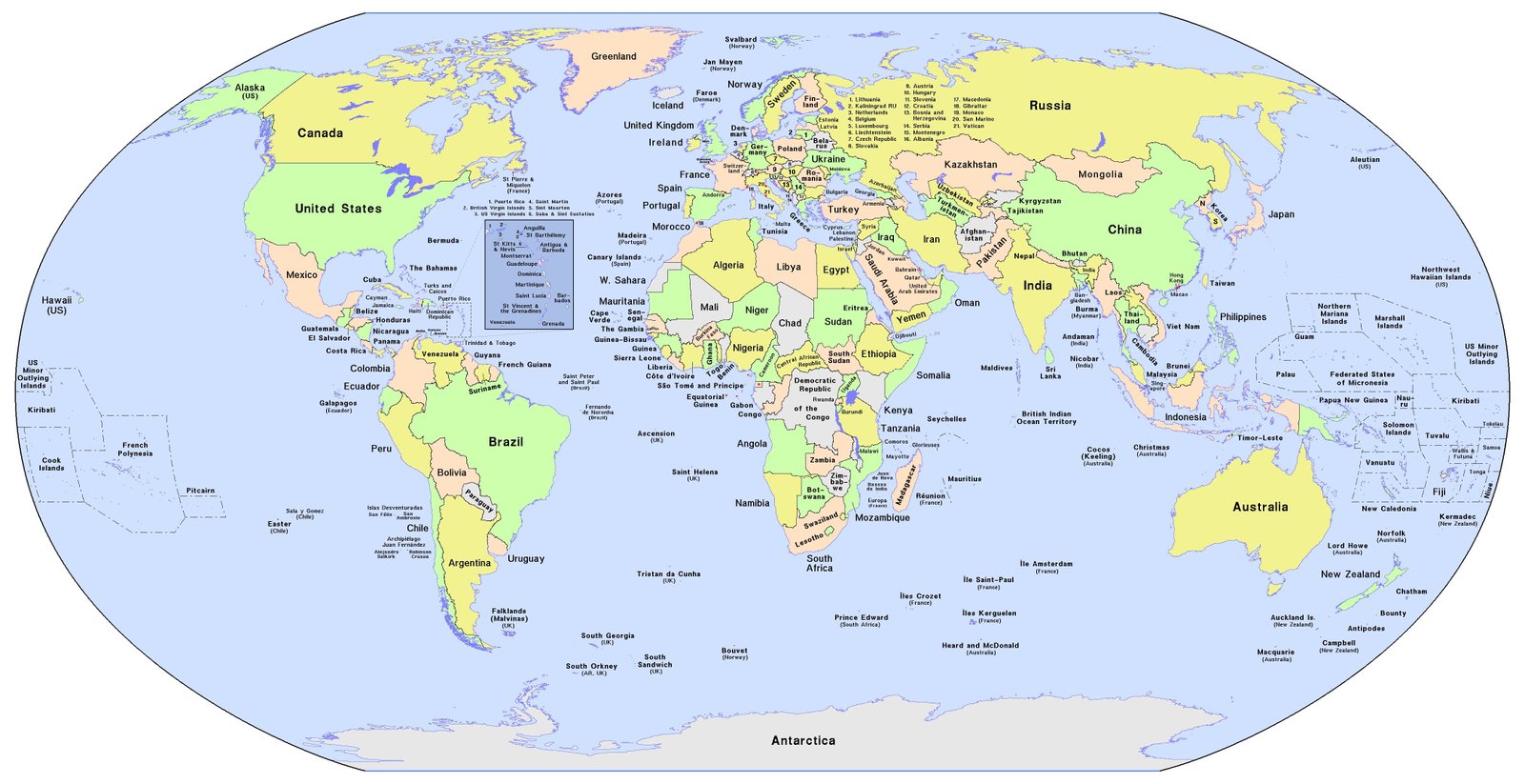
Solution